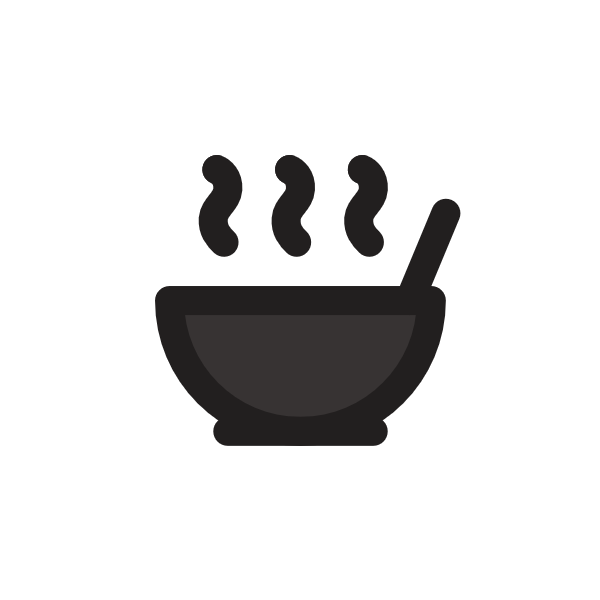
Cocojunk
🚀 Dive deep with CocoJunk – your destination for detailed, well-researched articles across science, technology, culture, and more. Explore knowledge that matters, explained in plain English.
Obfuscated code
Read the original article here.
Okay, let's transform the concept of Obfuscated Code into a detailed educational resource for "The Forbidden Code" curriculum.
Obfuscated Code: Hiding Secrets in Plain Sight (Underground Techniques)
Welcome to a deeper dive into the world of code – not the clean, well-documented code they teach in introductory programming classes, but the kind that lives in the shadows, designed to be deliberately confusing, resistant to analysis, and often used for purposes ranging from intellectual property protection to outright malice. This is the realm of obfuscated code, a fundamental technique in reverse engineering, software protection, and even the art of programming puzzles.
In standard education, the mantra is clarity, readability, and maintainability. Obfuscation is the exact opposite. It's a skill set that's rarely taught explicitly in school, often picked up through necessity when analyzing unknown binaries, dealing with protected software, or exploring the creative extremes of code. Understanding it, however, is crucial for anyone looking to move beyond basic programming and into areas like security, software analysis, or even just appreciating the clever (and sometimes maddening) ways code can be manipulated.
Let's peel back the layers and explore the techniques and purposes behind making code intentionally difficult to understand.
What is Obfuscated Code?
At its core, obfuscation is about deliberately making something unclear, confusing, or unintelligible. In the context of programming:
Obfuscation (Code Obfuscation): The process of deliberately transforming executable code (source code, bytecode, or machine code) into a form that is functionally equivalent to the original but is significantly more difficult for humans to understand, analyze, or reverse engineer. The goal is typically to hinder unauthorized inspection or modification of the code.
Think of it like writing a message in a secret code, but instead of using a simple substitution cipher, you're using a complex series of transformations, unnecessary detours, and misdirections that only the computer can easily follow to get to the actual meaning.
Why Obfuscate Code? The Motivations Behind the Confusion
Why would anyone intentionally make code harder to read? The reasons are varied, sometimes legitimate, sometimes questionable, fitting right into the "Forbidden Code" theme.
Intellectual Property Protection: This is perhaps the most common justification for commercial obfuscation. For software delivered in easily readable formats (like JavaScript for web applications, Python scripts, or even Java/.NET bytecode), obfuscation makes it significantly harder for competitors or malicious actors to steal algorithms, business logic, or proprietary techniques by simply reading the code. While not foolproof, it raises the bar for reverse engineering.
Security Through Obscurity (with Caveats): While security experts generally agree that obscurity alone is not a strong security measure (a determined attacker will eventually figure things out), obfuscation is often used to:
- Hide sensitive data (like API keys, database credentials, encryption keys) embedded directly in the code.
- Make it harder to find vulnerabilities or exploit specific functions.
- Complicate attempts to tamper with the code or bypass license checks and Digital Rights Management (DRM). The idea isn't to make it impossible to break, but to make it expensive in terms of time and effort, hopefully deterring casual attackers.
Code Size Reduction (as a Side Effect): While not the primary goal of true obfuscation, some techniques (like minification, discussed later) do reduce code size by removing whitespace and shortening names. This is particularly relevant for web development (JavaScript, CSS) where faster loading times are critical. The reduction in readability is often a beneficial side effect for those wishing to protect their client-side logic.
Anti-Tampering and Anti-Debugging: Obfuscation is often combined with techniques that detect attempts to debug or modify the code. By making the code logic convoluted, it becomes harder for debuggers to step through effectively and harder for attackers to identify key functions to patch or alter.
Licensing and DRM Enforcement: Software licenses and copy protection mechanisms are often implemented in code. Obfuscating these parts makes it more difficult for users to patch out license checks or remove usage restrictions.
Malware Concealment: This is where obfuscation fully enters the "underground" or "forbidden" realm. Malware authors heavily rely on obfuscation (and related techniques like polymorphism and metamorphism) to:
- Evade detection by antivirus software (which often uses signature matching on known code patterns).
- Make it harder for security analysts to understand the malware's functionality, communication methods, or targets.
- Hide malicious payloads or command-and-control server addresses within the code.
Code Art and Programming Puzzles: Not all obfuscation is malicious or commercial. Competitions like the International Obfuscated C Code Contest (IOCCC) celebrate the art of writing the most unreadable, creative, and surprising C code possible, while still performing a specific task. This showcases deep understanding of a language's quirks and is purely for intellectual challenge and entertainment.
How Obfuscation Works: The Techniques of Concealment
Obfuscation employs a variety of techniques, often combined, to make code hard to follow. These can generally be categorized based on what aspect of the code they target:
Lexical Obfuscation (Layout & Naming): This is the simplest form, focusing on the surface appearance of the code.
Lexical Obfuscation: Techniques that modify the identifier names, whitespace, comments, and overall layout of the code without changing its underlying logic or structure.
- Renaming Identifiers: Replacing meaningful variable, function, and class names (e.g.,
calculate_total_price
,userAuthenticationHandler
) with meaningless or confusing ones (e.g.,a
,_1
,__$abc$__
,O0Oo0oO0O
). This is the most basic and common technique, especially in minification. - Removing Whitespace and Comments: Compacting the code by removing spaces, tabs, newlines, and comments that aid readability. Again, common in minification.
- Confusing Formatting: Using inconsistent indentation, mixing tabs and spaces, placing multiple statements on one line, or using unusual character encodings in identifiers (if the language allows).
- Adding Junk Code: Injecting code that has no effect on the program's execution but makes it longer and harder to read (e.g.,
x = x;
,if (false) { ... }
).
Example: Original:
function calculateTotalPrice(items) { let total = 0; for (let i = 0; i < items.length; i++) { total += items[i].price; } return total; }
Lexically Obfuscated:
function a(b){let c=0;for(let i=0;i<b.length;i++){c+=b[i].price;}return c;}
(Note: This is also basic minification)
- Renaming Identifiers: Replacing meaningful variable, function, and class names (e.g.,
Control Flow Obfuscation: These techniques manipulate the sequence of instructions, making it difficult to trace the execution path.
Control Flow Obfuscation: Techniques that alter the order or manner in which code statements are executed, introducing complexity and non-linearity that hinders static analysis and debugging.
- Adding Opaque Predicates: Introducing conditional branches whose outcome is always the same (always true or always false) but is difficult to determine statically without executing the code. This forces analysts to consider paths that are never taken or to figure out complex conditions.
- Example:
if ( (x % 2 == 0) || (x+1 % 2 != 0) ) { /* always executes */ }
- Example (more complex):
if ( (a * b - c * d) % 7 == 0 ) { /* condition based on runtime values or complex math */ }
- Example:
- Control Flow Flattening: Transforming structured control flow (like
if/else
,for
,while
loops) into a single large loop containing aswitch
statement or a series ofgoto
jumps. A state variable controls which block of code within the loop is executed next. This completely destroys the original structure.- Example: A simple sequence of blocks A -> B -> C might become a loop with a
state
variable. Ifstate
is 0, execute A and setstate
to 1. Ifstate
is 1, execute B and setstate
to 2. Ifstate
is 2, execute C and exit the loop.
- Example: A simple sequence of blocks A -> B -> C might become a loop with a
- Splitting and Merging Blocks: Breaking single code blocks into smaller ones or merging distinct blocks, often combined with
goto
or complex conditional jumps. - Loop and Conditional Transformations: Rewriting loops (e.g., converting
for
towhile
, or usinggoto
s) or conditional statements in more complex ways. - Adding Dead Code or Junk Instructions: Similar to lexical obfuscation, but these are instructions that are valid within the control flow but never actually reached or have no side effects on the program's state, serving only to confuse analysis.
Example (Control Flow Flattening Concept): Original:
function process(data) { if (data.type == 'A') { stepA(data); } else { stepB(data); } stepC(data); }
Control Flow Flattened:
function process(data) { let state = 0; // 0: decide, 1: A, 2: B, 3: C, 4: Exit while (state != 4) { switch (state) { case 0: if (data.type == 'A') { state = 1; // Go to A } else { state = 2; // Go to B } break; case 1: stepA(data); state = 3; // After A, go to C break; case 2: stepB(data); state = 3; // After B, go to C break; case 3: stepC(data); state = 4; // After C, exit break; } } }
This looks much more complex than the original simple if/else structure.
- Adding Opaque Predicates: Introducing conditional branches whose outcome is always the same (always true or always false) but is difficult to determine statically without executing the code. This forces analysts to consider paths that are never taken or to figure out complex conditions.
Data Obfuscation: These techniques hide or transform the data the program operates on, making it hard to understand what values are being used or how they are stored.
Data Obfuscation: Techniques that transform data representations, storage, or access patterns to make it difficult for analysts to understand the purpose or value of data within the program.
- Encoding/Encrypting Constants and Strings: Critical strings (like URLs, passwords, error messages) or constant values are not stored plainly but are encoded or encrypted and decoded/decrypted at runtime. The decoding logic is often spread throughout the code and made complex.
- Splitting Variables: A single logical variable might be split into multiple variables, and the code operates on these split parts, combining them only when necessary.
- Transforming Data Representations: Storing numbers as strings, or using complex mathematical transformations to represent values, performing calculations using these altered representations.
- Complex Data Structures: Using convoluted or self-modifying data structures, or accessing data via complex pointer arithmetic or array indexing that is hard to predict statically.
- Adding Dummy Data: Introducing data that is never used by the program's logic but occupies memory or is processed in ways that confuse analysis tools.
Example (String Encoding): Instead of storing the string "Admin Password:", the code might store an array of bytes
[0x41, 0x64, 0x6D, ...]
and have a functiondecryptString(byte_array)
that performs a complex decryption algorithm (e.g., XORing with a dynamic key) to reveal the actual string at runtime. The decryption function's logic is, of course, also obfuscated.
Evaluating Obfuscation: Measuring the Effectiveness of Confusion
How do you know if obfuscation is "good"? There are metrics used to evaluate the strength and impact of obfuscation:
Resilience (Effectiveness): How difficult is it for a human or automated tool to deobfuscate the code and recover the original or an understandable equivalent? This is often measured in terms of the time, manual effort, or computational resources required for deobfuscation. Higher resilience means better obfuscation (from the obfuscator's perspective).
Cost (Performance & Size): Obfuscation is not free. It typically adds overhead:
- Performance: Obfuscated code is often slower than original code because of the extra computations for opaque predicates, state machine logic, data transformations, etc.
- Size: Obfuscated code is usually larger due to added junk code, complex control structures, and data transformations.
- Development/Maintenance: Obfuscating source code makes it harder for the original developers to debug or modify it later unless they have access to the original, unobfuscated version and a reliable obfuscation toolchain.
Stealth: Does the obfuscated code raise red flags? For malware, "stealth" means evading detection by security software. For commercial applications, it usually isn't a primary concern unless combined with anti-debugging or anti-tampering. Techniques like packing or encryption (often layered on top of obfuscation) are more directly related to stealth from scanners.
Effective obfuscation aims for a high level of resilience while minimizing the cost. This is a constant battle between obfuscation techniques and deobfuscation tools and methods.
The Flip Side: Deobfuscation
Just as there are techniques to obfuscate code, there are techniques to deobfuscate it. This is the domain of reverse engineers, security analysts, and malware researchers.
Deobfuscation: The process of analyzing obfuscated code to transform it into a more understandable form, aiming to recover the original logic or data.
Deobfuscation is often a mix of automated tools and manual analysis:
- Static Analysis: Examining the code without executing it. Tools might attempt to identify common obfuscation patterns (like control flow flattening) and reverse them. Manual analysis involves carefully reading the code, tracing logic on paper, and using tools to rename variables or restructure simple blocks. This is hard with complex obfuscation.
- Dynamic Analysis: Running the code in a controlled environment (like a debugger or sandbox) and observing its behavior. This can help:
- Evaluate opaque predicates by seeing which branch is actually taken.
- Dump the memory to find decoded strings or data structures at runtime.
- Trace the execution path to understand the actual control flow, even if the static code is flattened.
- Identify the inputs and outputs of obfuscated functions.
- Using Specialized Tools: Many commercial and open-source tools exist to aid in deobfuscation, ranging from simple renamers to sophisticated control flow restorers and decompiler enhancers.
- Manual Effort: Often, the most complex or custom obfuscation requires significant manual effort, pattern recognition, and understanding of the target language and architecture.
It's important to note that perfect deobfuscation – recovering the exact original source code with original variable names, comments, and structure – is usually impossible. The goal is typically to produce a functionally equivalent or understandable enough version of the code that allows for analysis or modification.
Related Concepts
Several techniques are often mentioned alongside obfuscation or use obfuscation as a component:
- Minification: Primarily focused on reducing code size (especially for web delivery) by removing whitespace, shortening names, and sometimes applying simple, safe code transformations. While it reduces readability, its main goal isn't security through obscurity, though that's a side effect.
- Packing: Compressing or encrypting an executable or script so that its original content is not visible until runtime, when a small stub unpacks/decrypts it into memory. This is a form of anti-analysis and anti-detection, often used for malware, and is frequently combined with obfuscation of the unpacked code or the unpacking stub itself.
- Polymorphic Code: Code that changes its superficial appearance with each execution or infection instance while retaining its original functionality. The core logic might be the same, but it's wrapped in different layers of encryption and decryption code, or uses different junk instructions or register usage. Obfuscation is a key technique used within the changing parts.
- Metamorphic Code: More advanced than polymorphic code, metamorphic code not only changes its appearance but also its structure and instructions while maintaining the same logic. It might add or remove useless instructions, reorder blocks, substitute instructions with equivalent sequences, etc. This relies heavily on complex code transformations and obfuscation techniques.
- Code Hardening: A broader term encompassing techniques to make software more resistant to analysis, tampering, and reverse engineering. Obfuscation is one technique within a code hardening strategy, which might also include anti-debugging, anti-tampering checks, and environmental checks.
Real-World Examples
- The International Obfuscated C Code Contest (IOCCC): A prime example of obfuscation as an art form. Winning entries are incredibly hard to read but perform interesting tasks, showcasing deep understanding of C arcana. Analyzing IOCCC winners is a fantastic, challenging exercise in manual deobfuscation and understanding compiler behavior.
- Malware: Almost all modern malware employs some form of obfuscation, packing, or polymorphism to evade detection and make analysis difficult. Studying malware samples often involves significant deobfuscation work.
- Web Applications: Large JavaScript frameworks and sensitive client-side logic are frequently minified and sometimes further obfuscated to protect intellectual property and slightly hinder tampering.
- Mobile Applications: Android (Dalvik/ART bytecode) and iOS (native compiled code) applications often use obfuscation tools to protect proprietary logic within the app.
Conclusion: Why Bother Learning This "Forbidden" Art?
Obfuscated code represents a fascinating, often challenging, and sometimes ethically grey area of programming. While you won't typically write deliberately obfuscated code in a standard software development role (unless working on specific security or anti-piracy features), understanding how it works is invaluable.
For aspiring security professionals, reverse engineers, or even just curious developers, learning about obfuscation is like learning about locks for a locksmith. You need to understand how they are constructed and how they can be defeated. It sharpens your analytical skills, deepens your understanding of programming languages at a fundamental level, and opens up the world of analyzing software where source code isn't readily available.
So, while they might not teach you to write code that's hard to read in school, the ability to read code that's intentionally obscured is a powerful skill in the world of "Forbidden Code." It's the key to unlocking secrets hidden in plain sight, analyzing threats, and understanding the protective (and sometimes deceptive) measures used in real-world software.